Application Programming Interface (API) creation is taking connections between different software programs so they can talk to each other and do things. In today’s digital world, software engineers and writers must know how to create APIs. APIs make it possible to build flexible, open, and efficient systems, letting different apps and services work together without any problems.
The best ways to learn how to do API development are covered in this blog. We’ll look at key ideas, methods, and real-life cases to help you learn everything from the basics of APIs to more advanced techniques like RESTful and GraphQL architectures. Readers will learn a lot about the details of API development and be able to make APIs that are strong, safe, and ready for the future by the end.
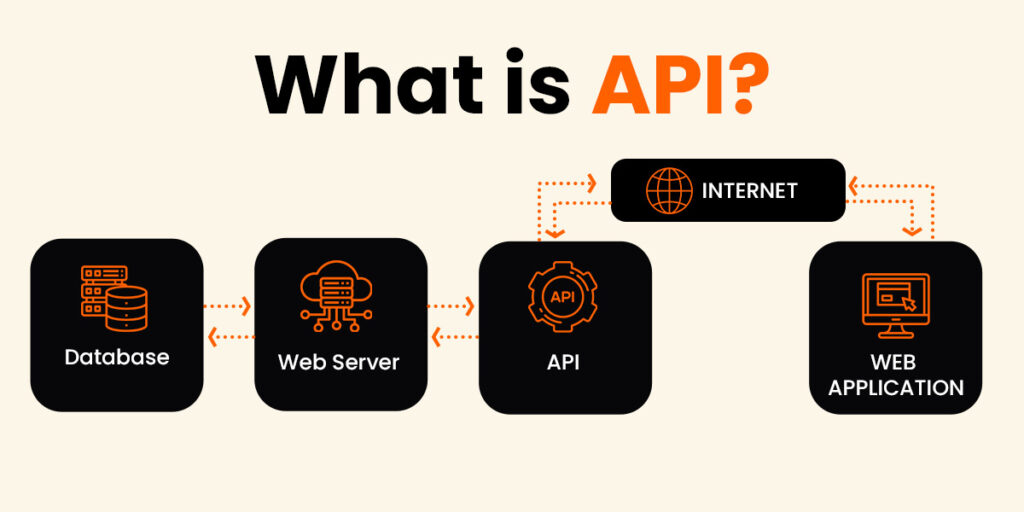
How to Understand APIs
What does an API mean?
There is a way for different software programs to talk to each other called an Application Programming Interface (API). It describes a set of rules, standards, and tools for making software and ensuring all its parts work together smoothly. Simply put, an API tells software components how to work together so that makers can use features or data from one app in another.
Different kinds of APIs
APIs come in different types, each of which is best for different situations and uses. Some common types are:
- Web APIs let you use the internet to get to web-based services.
- Library APIs let you use certain methods or classes in a program library.
- Operating System APIs make it easier for programs to talk to the operating system underneath, which lets programs use system resources.
Why APIs are important for making new apps
APIs are important to current software development because they allow for collaboration, scaling, and extension. They make it easier for coders to use functions that are already there, connect different systems, and make complicated apps faster. APIs also encourage teamwork and new ideas by making it easier for software components and services to share data and services. Overall, APIs give developers the tools they need to make strong, feature-packed apps that meet the changing needs of businesses and users in the digital age.
API design that is consistent and clear.
API design is based on consistency and clarity, making APIs easy for coders to understand and use. Developers can make APIs easier to understand and predict using uniform name rules, argument forms, and answer structures. This makes the code easier to read and speeds up the learning process for coders who use the API.
Separate versions and backward compatibility
Versioning is an important part of API development because it helps keep old connections working while adding new features or changes. Using a versioning approach like semantic versioning, developers can make API changes clear and ensure that current clients can still use it even as it changes.
Taking care of mistakes with grace
Handling errors is an important part of making an API that works well. APIs should send error messages and HTTP status codes that make sense so coders can quickly figure out what’s wrong and fix it. Also, error replies should use the same forms and standards at all locations to make handling errors easier for client apps.
Concerns about security
When making APIs, security is very important to keep private data safe and stop people from getting in without permission. To control who can access API resources, developers should set up login and permission tools like OAuth or API keys. APIs should also be made with security best practices in mind, such as validating input, encrypting it, and protecting against common security holes like SQL injection and cross-site scripting (XSS).
Documentation and making APIs easy to find
For API acceptance and use to go smoothly, detailed guidance is a must. Developers should give clear and brief guidance that includes examples of how to use the API, locations, request and answer forms, and login methods. Developers can also use API development tools like Postman or Swagger to make live instructions and APIs easier to find. Documentation can make the merging process easier for developers and help more developers use the API by giving it more importance.
Tips for Making API Development Go More Smoothly
Making the right choice between computer language and framework
When building an API, choosing the right computer language and platform is important because it affects speed, scaling, and worker productivity. Consider the project’s unique needs, the ecosystem’s growth, and the community’s support. For example, Python is used a lot because it is easy to use and read, while Java is used because it is reliable and can grow. Frameworks like Flask for Python or Spring Boot for Java can speed up development and handle common jobs like routing, serialization, and security with built-in features.
Using design standards to make things scalable and easy to manage
Design patterns are tried-and-true ways to solve common design issues. They help make API development more scalable, maintainable, and code-reusable. Patterns like Model-View-Controller (MVC) or RESTful design principles help order and break down APIs into separate modules. By using these patterns, developers can keep different issues separate, encourage components to work with each other without being tightly connected, and make it easier to maintain and change the API over time.
Tests that are run automatically and continuous integration
Continuous integration (CI) and automated testing are important ways to ensure that APIs are stable and reliable throughout development. Unit tests check the functionality of individual parts, and integration tests check how well different parts of the API work together. If you add these tests to a CI/CD process, they will be run automatically every time the script is changed. This way, bugs, and regressions will be found quickly.
Implementing cache techniques to improve speed
Caching can make APIs much faster and more efficient by cutting down on the delay caused by repeated or resource-intensive processes. You can cache different data types, like database query results, API replies, or calls to external services. You can do this with in-memory or global caching with Redis or Memcached. APIs can reduce reaction times and improve scaling by saving often-used data or processes. This is especially helpful when there is a lot of traffic or load.
Keep an eye on and analyze API health.
Monitoring and analytics are very important for keeping APIs in live settings healthy and running well. Keep an eye on important measures like speed, mistake rates, and response times with tracking tools like Prometheus or Datadog. Use logging and tracking to learn more about how APIs work and quickly find problems. By monitoring API speed and usage trends, developers can find slow spots, make the best use of resources, and ensure users have a smooth experience. Also, use data to learn about how APIs are being used, how users are behaving, and how to improve speed so that you can make smart decisions and keep getting better.
Making RESTful APIs
How RESTful architecture works
Representational State Transfer, or REST, is a way of building apps that work over networks. RESTful APIs follow rules that encourage ease, flexibility, and the ability to work with other systems. One of these ideas is statelessness, which means that every request from a client to a server has all the information the server needs to understand and carry out that request. RESTful APIs also use standard methods like HTTP to communicate, which can be used on various systems and devices.
Rules for naming resources
URIs (Uniform Resource Identifiers) stand for resources in RESTful API design. Following the right name standards is key to making APIs easy to use and understand. Resource URIs should be organized and show how resources are related. Nouns, not verbs, should be used to describe things. For instance, “/users” is a group of users, while “/users/{id}” is a single person whose unique ID can be found.
How do you use HTTP and result codes?
Following the CRUD (Create, Read, Update, Delete) ideas, RESTful APIs use HTTP methods (GET, POST, PUT, DELETE, etc.) to do things with resources. Each HTTP way does a different thing: GET gets images of resources, POST makes new resources, PUT changes current resources, and DELETE gets rid of resources. Additionally, HTTP status codes tell you what happened with a request by showing whether it was successful, unsuccessful, or something else, like a return or a client error.
Taking care of permission and authentication
Authorization and authentication are important to keeping RESTful APIs safe and managing who can access resources. Clients who want to use the API are authorized to make sure they are who they say they are. Authorization then decides what rights to give authenticated clients. API keys, OAuth tokens, and JSON Web Tokens (JWT) are all common ways to authenticate users. Permission can be done with role-based access control (RBAC), attribute-based access control (ABAC), or other permission systems. RESTful APIs make sure that only allowed users can access and change resources by using login and permission methods. This protects the privacy and security of the data.
Using GraphQL to make APIs more flexible
A Quick Look at GraphQL
As an option to standard RESTful APIs, Facebook created GraphQL, a query language for APIs meant to be faster and more flexible. GraphQL lets clients say exactly what data they need, making it easier to get the data they need quickly.
Pros compared to RESTful APIs
There are several reasons why GraphQL is better than RESTful APIs, such as
- Less info being over-fetched and under-fetched
- One location for all requests for data
- A strongly typed structure makes it easier for developers to work with
- The ability to get more than one item with a single question
- Automatic creation of documents based on defined schemas
Putting GraphQL APIs into action
To use GraphQL APIs, you need to create a model that lists the available data types and actions that can be done on them. The developers then make resolvers to handle new changes and questions. These resolvers get data from the right sources and prepare the answer based on the client’s request.
How can GraphQL be used?
GraphQL works especially well in situations where freedom and speed are very important, like –
- Mobile apps that need different amounts of data
- Web apps have complex needs for getting info.
- Microservices designs need to combine data from several different sources.
In apps like chat rooms and social networking sites, getting and changing info in real-time.
Best Practices for API Security
Mechanisms for authentication
The authentication process ensures only authorized people or systems can access the API. API keys, OAuth (Open Authorization), and JSON Web Tokens (JWT) are all common ways to prove who you are. These systems ensure clients are who they say they are and let them use approved resources.
Controlling entry and giving permission
Authorization decides what rights are given to people or systems that have been verified. Tools for controlling access, like role-based access control (RBAC) or attribute-based access control (ABAC), ensure that users follow specific rules based on their jobs or traits. This ensures that users can only use the resources they are allowed to handle.
Validation of input and cleaning of data
Validating input and cleaning up data is crucial for stopping injection attacks and other security holes. APIs should check and clean input data to ensure it follows the right standards and has no harmful content. Because of this, cross-site scripting (XSS), SQL attacks, and other common security threats are less likely to happen now.
Protocols for encryption and safe contact
Using secure communication methods, like HTTPS (HTTP Secure), to encrypt private data and encrypt data in transit keeps it safe from being listened to or changed. Transport Layer Security (TLS) ensures that clients and servers can talk to each other safely, keeping private data from getting into the wrong hands. APIs protect data privacy and security by securing data and enforcing secure communication methods. This lowers the risk of data leaks and illegal access.
Testing and writing API documentation
Why detailed recording is important?
They need detailed instructions to make it easier for people to accept and use APIs. It tells developers exactly how to use the API by listing routes, request and answer forms, login methods, and examples of how to use the API. Well-documented APIs make it easier for developers to learn how to use them, speed up interaction, and make the whole experience better for developers.
Frameworks and tools for writing documentation for APIs
Many tools and platforms can be used to describe APIs, making the process and documentation easier to read. Many developers like Swagger, OpenAPI, and API Blueprint because they let them make live data from API specs. Some of the things that these tools can do are automatically make API reference documents, let you explore APIs interactively, and generate client code.
APIs should be tested both separately and as a whole.
Unit testing and integration testing are very important for ensuring that APIs are reliable. Unit tests check how different parts or features of the API work together, while integration tests check how different parts of the API work together. By making testing processes automatic and adding them to a continuous development chain, developers can find bugs quickly, stop regressions, and keep the API stable.
Exploratory testing and making fake APIs
To find possible problems or edge cases that automatic tests might not cover, exploratory testing involves trying the API by hand. Also, API mocking tools let writers fake API answers and actions, which lets them try different situations without using real server services. Developers can ensure all tests are covered, and the API is better overall by using both exploratory testing and API mocking.
Conclusion
To get good at API programming, you must follow best practices like ensuring the design is consistent, using strong security measures, and writing detailed documents. Scalable and effective APIs can be made using fast computer languages, design patterns, and testing methods.
In the area of API development, which is always changing, it’s important to keep learning and getting better. Developers can improve their skills and make APIs that meet the needs of modern software development by keeping up with the latest technologies, tools, and industry trends.
It’s very important to know how to create APIs in today’s digital world, where everything is linked. Software collaboration depends on APIs, which make it possible for different systems to talk to each other easily and encourage new ideas. By learning API development, developers can make software apps more efficient, scalable, and interoperable. Ultimately, this helps advance technology and bring about digital change across all industries.
What do you think?
It is nice to know your opinion. Leave a comment.