Fast Track Overview
In this essential guide, we’ll walk you through the process of installing Node.js and NPM (Node Package Manager) on both Windows and Mac systems. Node.js is a versatile, open-source JavaScript runtime built on Chrome’s V8 engine, enabling developers to build high-performance, scalable web applications. NPM, on the other hand, is the package manager for Node.js, providing access to a vast repository of open-source libraries and tools. Whether you’re a beginner or an experienced developer, this guide will help you get set up quickly so you can start leveraging the power of Node.js and NPM for your projects.
What is Node.JS?
Node.js is a powerful, cross-platform, open-source JavaScript runtime environment, capable of running on Windows, Linux, Unix, macOS, and more. Released in 2009 by Ryan Dahl, it has become a popular tool for building both front-end and back-end applications. With Node.js developers can use JavaScript to create scalable, high-performance projects for any platform.
Why Node.JS for Web Application Development?
Node.js is the best choice for web application development because of its many benefits, including:
Fast & Scalable
Node.js uses an event-driven, non-blocking I/O model, making it highly efficient and scalable for web applications, handling multiple connections simultaneously without compromising speed or performance.
Productivity
With JavaScript on both the front-end and back-end, Node.js enhances developer productivity by allowing code reusability and a consistent development environment, leading to faster project delivery.
High Performance
Node.js uses Google’s V8 JavaScript engine, which compiles JavaScript into machine code, improving application speed and ensuring faster execution, making it ideal for performance-critical applications.
Easy to Learn
JavaScript’s widespread usage makes Node.js easy to learn for most developers. Those familiar with JavaScript can quickly adopt Node.js for both front-end and back-end development.
Error Handling
Node.js offers robust error-handling mechanisms, providing clear error messages and facilitating efficient debugging processes, resulting in more stable and reliable applications.
Cost-Effectiveness
Using JavaScript for both client-side and server-side reduces the need for separate developer teams, lowering costs and simplifying the development methodology.
Active Community Support
Node.js has a vast and active community of developers, offering extensive libraries, tools, and frameworks, which provide solutions to common development challenges and contribute to faster problem-solving.
Cross-Platform Development
Node.js supports cross-platform development, allowing developers to build applications for various operating systems like Windows, Linux, and macOS without the need for separate codebases.
Getting Started with Node.JS
1. Installation
To begin, you’ll need to install Node.js on your system. You can download the latest version from the official Node.js website. The installation package includes both Node.js and npm (Node Package Manager), which you’ll use to manage your project dependencies.
2. Setting up Your First Project
Once Node.js is installed, create a new directory for your project and navigate into it via the terminal:
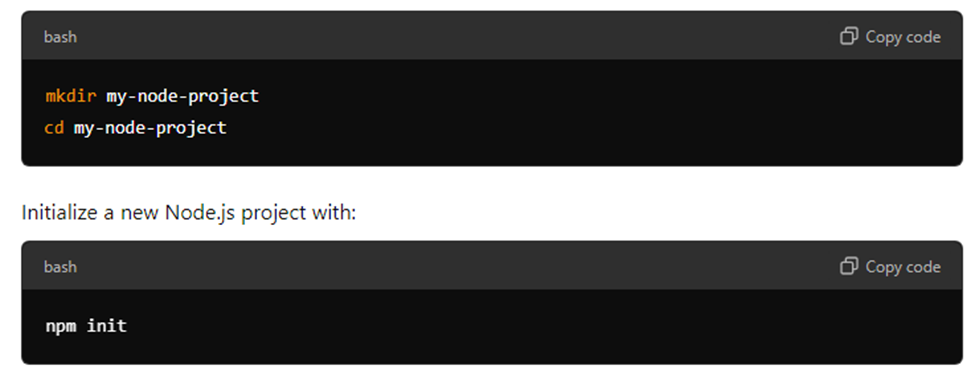
This command will prompt you to enter details about your project and generate a package.json file, which manages project dependencies and scripts.
3. Creating Your First Script
Create a new file named app.js in your project directory. This will be your main application file. Open app.js in a text editor and add the following code:
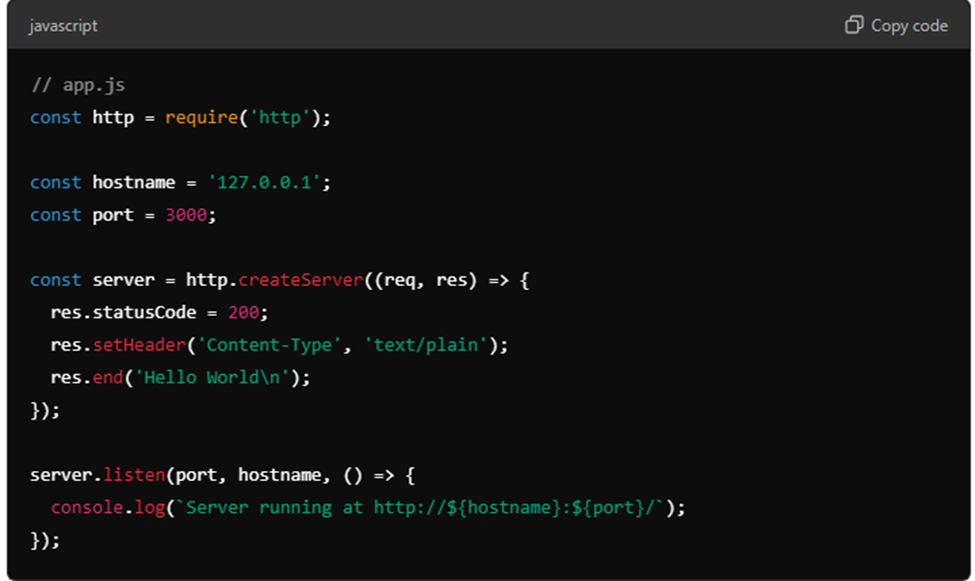
This simple script creates a basic HTTP server that responds with “Hello World” to any request.
4. Running Your Application
Run your Node.js application by executing the following command in your terminal:

You should see a message indicating that the server is running. Open a web browser and navigate to http://127.0.0.1:3000 to see the “Hello World” message.
5. Exploring Node.js Modules
Node.js comes with a set of built-in modules (like http, fs, path, etc.) that provide various functionalities. Additionally, you can install third-party modules from npm to extend the capabilities of your application. For example, to install the popular express framework, use:

Then, you can use it in your application like so:
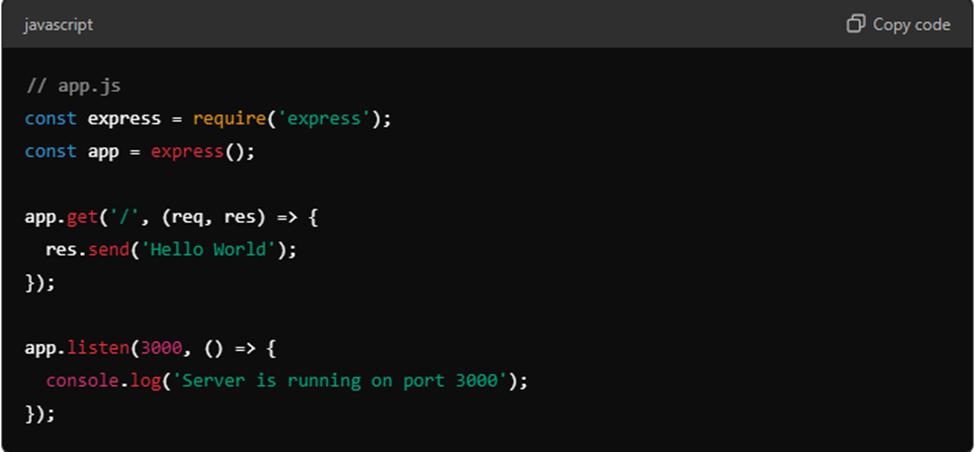
6. Learning More
To advance your Node.js skills, explore additional resources such as the Node.js documentation, online tutorials, and community forums. Building real-world applications and contributing to open-source projects can also enhance your expertise.
Getting started with Node.js opens up a world of possibilities for building server-side applications and services. With its vast ecosystem and supportive community, Node.js is a versatile tool for modern web development.
What is NPM? Exploring Its Definition and Purpose
NPM (Node Package Manager) is a powerful tool used for managing libraries and dependencies in JavaScript projects, especially with Node.js. It allows developers to easily install, update, and manage reusable code packages, streamlining the development process and promoting collaboration across projects by sharing modules.
Use of NPM Along with Node.JS
Package Management: NPM helps install, update, and manage libraries and dependencies required for Node.js projects.
Dependency Resolution: Automatically resolves and manages project dependencies, ensuring compatibility and avoiding conflicts.
Version Control: NPM allows tracking and controlling specific versions of packages, ensuring consistency across environments.
Script Automation: Enables the execution of custom scripts for automating repetitive tasks like testing, building, and deployment.
Code Sharing: Facilitates sharing of reusable code modules across different projects and teams.
Community Packages: Provides access to a large repository of open-source packages, speeding up development with pre-built solutions.
How to Install Node JS and NPM in Windows?
Node.js can be installed on Windows using various methods. The choice of approach may depend on the existing development environment. Below are the common methods for installing Node.js, complete with steps and screenshots.
Method 1: Using the Node.js Installer
1. Download the Installer
- Visit the official Node.js website.
- Choose the Windows Installer (.msi) for your system architecture (32-bit or 64-bit).
2. Run the Installer
- Double-click the downloaded .msi file to start the installation.
- Click “Next” to proceed.
3. Accept the License Agreement
- Read and accept the license agreement. Click “Next.”
4. Select Installation Folder
- Choose the destination folder or keep the default path. Click “Next.”
5. Install Node.js
- Click “Install” to begin the installation process.
- Once completed, click “Finish.”
6. Verify Installation
- Open Command Prompt and run node -v and npm -v to check the installed versions.
Method 2: Compiling from Source
1. Download Source Code
- Go to the Node.js GitHub repository.
- Download the source code as a ZIP file or clone the repository.
2. Install Build Tools
- Install necessary build tools and dependencies using tools like Windows Build Tools or Visual Studio.
3. Compile and Install
- Extract the source code and open Command Prompt.
- Navigate to the extracted folder and run Python configure followed by Ninja to build the application.
- Run make install to complete the installation.
4. Verify Installation
- Similar to Method 1, use node -v and npm -v to verify the installation.
- By following these methods, you can successfully install Node.js on your Windows system, tailored to your development needs.
How to Install Node JS and NPM in Mac?
If you’re using macOS, you can easily install Node.js and NPM using the .pkg installer. Follow these steps for a smooth installation process:
Step 1: Download the .pkg Installer
1. Visit the Official Website
- Go to the Node.js official website.
- On the download page, select the macOS Installer (.pkg) for your system.
2. Choose the Right Version
- You’ll typically find two versions: LTS (Long Term Support) and Current-v21.0.0. Choose the version that suits your needs.
Step 2: Run Node.js Installer
1. Open the .pkg File
- Locate the downloaded .pkg file in your Downloads folder and double-click to start the installation.
2. Follow the Installation Wizard
- Click “Continue” and then “Agree” to accept the license agreement.
- Choose the installation location or proceed with the default path.
- Click “Install” and enter your administrator password if prompted.
3. Complete the Installation
- Once the installation is complete, click “Close” to exit the installer.
Step 3: Verify Node.js Installation
1. Open Terminal
Launch the Terminal application from your Applications > Utilities folder.
2. Check Node.js Version
- Run the command node -v to verify that Node.js is installed correctly. You should see the version number of Node.js.
Step 4: Update Your NPM Version
1. Check the Current NPM Version
- In Terminal, run npm -v to see the current version of NPM.
2. Update NPM
- To ensure you have the latest version of NPM, run npm install -g npm@latest in Terminal.
By following these steps, you’ll have Node.js and NPM installed and up-to-date on your macOS system, ready for development.
Key Consideration to Node JS and NPM Installation
When installing Node.js and NPM (Node Package Manager), several key considerations ensure a smooth and effective setup:
Choose the Right Version
Stable vs. LTS
Opt for the Long-Term Support (LTS) version of Node.js if you seek stability and reliability for production environments. The latest stable version may offer new features but could be less tested.
Compatibility
Ensure that the Node.js version is compatible with your project requirements and any existing dependencies.
Verify System Requirements
Operating System
Confirm that your operating system (Windows or macOS) is supported by the Node.js version you plan to install.
Hardware Specifications
Ensure your system meets the hardware requirements for optimal performance of Node.js applications.
Choose an Installation Method
Installer
Use the official Node.js installer for your operating system to simplify the setup process.
Package Managers
On Unix-based systems, consider using package managers like apt (for Debian/Ubuntu) or brew (for macOS) for installation.
NPM Installation
Bundled with Node.js
NPM is typically bundled with the Node.js installer, but verify its version after installation using npm -v to ensure it’s up-to-date.
Global vs. Local Packages
Understand the difference between globally and locally installed packages to manage dependencies effectively.
Post-Installation Verification
Node.js and NPM Versions
Check the installation by running node -v and npm -v in your terminal to confirm that both are correctly installed and accessible.
Test Environment
Run a basic Node.js script or install a package to ensure that your environment is set up correctly.
Ending Lines: Installation of Node.js and NPM on Windows and Mac
In this blog, we provide a step-by-step guide for installing Node.js and NPM on Windows and Mac. We start by explaining how to download and install the Node.js installer from the official website for both operating systems. On Windows, we use the executable installer, while on Mac, we utilize the .pkg installer. The blog includes code examples and screenshots to illustrate each step clearly.
We also cover the verification process, showing how to check the installed versions of Node.js and NPM using terminal commands. Additionally, we explain why Node.js is a popular choice for web development, highlighting its asynchronous, event-driven architecture that enhances performance and scalability. By following this guide, you’ll have Node.js and NPM set up and ready to streamline your development workflow.
What do you think?
It is nice to know your opinion. Leave a comment.